How to use INT 13H Extensions in C Programming
We can call the Extension Functions of INT 13H with the same C functions (int86(), int86x() etc.) , which we were using in the earlier chapters. Let us learn it by an example.
The following example gives the focus to the three functions (Check Extensions present, extended read and extended write). However we are not going to use extended write function in this chapter.
The program first checks if extensions are supported or not if the extensions are present for INT 13H, It reads the absolute sector 0 (thus MBR) of the disk. The coding of the program proceeds in the following manner:
/* Program to access sectors beyond 8.46 GB using INT 13 BIOS extensions */
#include<stdio.h>
#include<dos.h>
/* Assigns the identifier to the data type */
typedef unsigned char Byte;
typedef unsigned int Word;
typedef unsigned long DWord;
/* disk_packet structure is loaded in DS:SI and command
executed */
struct disk_packet
{
Byte size_pack; // Size of packet must be 16 or 16+
Byte reserved1; // Reserved
Byte no_of_blocks;// Number of blocks for transfer
Byte reserved2; // Reserved
/* Address in Segment:Offset format */
Word offset; //offset address
Word segment; //segment address
/* To Support the Disk Even of Capacity of
1152921504.607 GB */
DWord lba1;
DWord lba2;
}disk_pack;
/* Function to check if the Extensions are supported */
void check_ext_present()
{
union REGS inregs, outregs; /* Input Registers and
Output */
inregs.h.ah=0x41; /* Function to Check
Extension Present */
inregs.x.bx=0x55AA;
inregs.h.dl=0x80; /* Drive No for first Hard Disk */
int86(0x13,&inregs,&outregs); /*Call interrupt */
if(outregs.x.cflag)
{
/* Extension Not Supported */
printf("\nBios extension not supported");
exit(1);
}
if(outregs.x.bx==0xAA55)
if(outregs.x.cx & 0x1)
/* Extension Present */
printf("\nExtended I/O supported");
}
/* Function to read the Sector */
void read_sectors(void *buffer)
{
union REGS inregs, outregs; /* Input and Output
Registers */
struct SREGS segregs; // Segment Registers
disk_pack.size_pack=16; // Set size to 16
disk_pack.no_of_blocks=1; // One block
disk_pack.reserved1=0; // Reserved Word
disk_pack.reserved2=0; // Reserved Word
disk_pack.segment=FP_SEG(buffer);// Segment of buffer
disk_pack.offset=FP_OFF(buffer); // Offset of buffer
/* request for MBR of hard disk 1 */
/* Read Absolute sector 0 */
disk_pack.lba1=0; /* LBA address, Contains first
32 bits */
/* We normally require( drives < 2.1 T.B) to set
only this */
disk_pack.lba2=0; // Last 32 bit address
inregs.h.ah=0x42; // Function to read
inregs.h.dl=0x80; // Drive Number for First Hard Disk inregs.x.si = FP_OFF(&disk_pack); /*Make DS:SI point
to disk_pack */
segregs.ds = FP_SEG(&disk_pack);
/* Call Interrupt */
int86x(0x13,&inregs,&outregs,&segregs);
if(outregs.x.cflag)
printf("\n\nError %d\n",outregs.h.ah);
else
printf("\n\nI hope Everything is all Right");
}
//// Write Sector Function \\\\
void write_sector()
{
/* It will be written in the same way as read_sectors function, except Function Number, which will be 0x43 in this function. We’ll discuss extended write function in next chapters.
Before Using this Function please check and verify what are you going to do. You should have the proper knowledge of what you are going to do. Use of this function in lack of knowledge or carelessly may destroy your data. */
}
/// Main Function \\\\
void main()
{
int i=0;
/* buffer to hold MBR */
Byte mbr[512];
clrscr();
/* check for BIOS extension present */
check_ext_present();
/* read sector */
read_sectors(&mbr);
printf("\n\n Data of MBR \n");
printf("▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀▀\n\n\n");
while(i++<512)
{
/* display the MBR buffer */
printf("%c",mbr[i]);
}
}
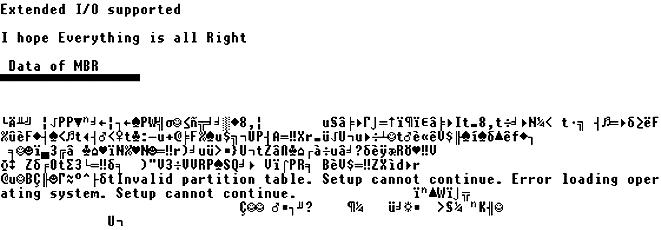
Comments on Coding of the program
typedef Assigns the identifier to the data type such that typedef unsigned char Byte; assigns identifier Byte for the data type char. Similarly identifier Word is assigned to int and DWord is assigned to long.
The disk_packet structure is loaded in DS:SI and the command (such as extended read, extended write or verify etc.) is executed. See the functions of IBM/MS INT 13H Extensions (Function 41H to Function 49H) given before.
The check_ext_present() function checks if the extensions are available/supported or not. The function is called with BX=55AAH (inregs.x.bx=0x55AA;) and if extensions are supported the BX register is set to AA55H. (See Function 41H given before)
The Function read_sectors is used to read the absolute sector of the disk, specified by disk_pack.lba1. In this program we have given disk_pack.lba1=0, thus we are going to read the absolute sector 0 (See the Note Below) thus going to read the MBR of the disk.
The write_sector function is also same as the read_sectors function and will be written in the same way but with the different Function options. We shall use it in the next chapters.
Note:
We read the disk sectors in the following two ways:
- Relative Sector Read (or Write)
- Absolute Sector Read (or Write)
In Relative Sector Read we read the disk sectors in accordance with CHS (Cylinder, Head and Sector) geometry of the disk. In relative sector read the MBR of the disk (First Sector of the disk) is on Cylinder 0, head 0 and Sector 1.
In the Absolute reading of the disk sectors, we need not to specify the Cylinder or Head numbers in our program. The absolute sectors are counted from absolute sector 0.
Thus if we are going to read the MBR of the disk (First sector of the disk), we are going to read absolute sector 0. It is the work of BIOS to convert the absolute sector number to its corresponding Cylinder, Head and Sector number.
As in absolute sector reading (or writing), we have to calculate only the absolute sectors within the loop in the operations such as entire disk reading or writing, whereas in case of relative sector reading (or writing), we need to run three loops at a time for calculating CHS therefore absolute sector reading/writing is much faster than relative sector reading/writing.
For example, if we have any hard disk with the 16 heads (sides), 12 Cylinders and with 63 sectors, the table given next, shows the procedure and difference of both the reading methods and thus shows how absolute sector approach may make our time taking programs (such as entire disk reading/ writing or entire disk wiping programs etc.) to run much faster:
Relative Sectors Reading |
Absolute Sector reading |
Cylinder =0, Head =0, Sector = 1 |
Absolute Sector = 0 |
Cylinder =0, Head =0, Sector = 2 |
Absolute Sector = 1 |
Cylinder =0, Head =0, Sector = 3 |
Absolute Sector = 2 |
.
.
.
. |
.
.
.
. |
Cylinder =0, Head =0, Sector = 62 |
Absolute Sector = 61 |
Cylinder =0, Head =0, Sector = 63 |
Absolute Sector = 62 |
Cylinder =0, Head =1, Sector = 1 |
Absolute Sector = 63 |
Cylinder =0, Head =1, Sector = 2 |
Absolute Sector = 64 |
Cylinder =0, Head =1, Sector = 3 |
Absolute Sector = 65 |
Cylinder =0, Head =1, Sector = 4 |
Absolute Sector = 66 |
.
.
.
. |
.
.
.
. |
Cylinder =0, Head =1, Sector = 63 |
Absolute Sector = 125 |
Cylinder =0, Head =2, Sector = 1 |
Absolute Sector = 126 |
Cylinder =0, Head =2, Sector = 2 |
Absolute Sector = 127 |
Cylinder =0, Head =2, Sector = 3 |
Absolute Sector = 128 |
.
.
.
. |
.
.
.
. |
Cylinder =0, Head =15, Sector = 63 |
Absolute Sector = 1007 |
Cylinder =1, Head =0, Sector = 1 |
Absolute Sector = 1008 |
Cylinder =1, Head =0, Sector = 2 |
Absolute Sector = 1009 |
Cylinder =1, Head =0, Sector = 3 |
Absolute Sector = 1010 |
.
.
.
. |
.
.
.
. |
Cylinder =1, Head =0, Sector = 63 |
Absolute Sector = 1070 |
Cylinder =1, Head =1, Sector = 1 |
Absolute Sector = 1071 |
Cylinder =1, Head =1, Sector = 2 |
Absolute Sector = 1072 |
Cylinder =1, Head =1, Sector = 3 |
Absolute Sector = 1073 |
.
.
.
. |
.
.
.
. |
Cylinder =1, Head =15, Sector = 63 |
Absolute Sector = 2015 |
Cylinder =2, Head =0, Sector = 1 |
Absolute Sector = 2016 |
Cylinder =2, Head =0, Sector = 2 |
Absolute Sector = 2017 |
Cylinder =2, Head =0, Sector = 3 |
Absolute Sector = 2018 |
.
.
.
. |
.
.
.
. |
Cylinder =11, Head =15, Sector = 60 |
Absolute Sector = 12092 |
Cylinder =11, Head =15, Sector = 61 |
Absolute Sector = 12093 |
Cylinder =11, Head =15, Sector = 62 |
Absolute Sector = 12094 |
Cylinder =11, Head =15, Sector = 63 |
Absolute Sector = 12095 |
The information of partition table of MBR, which is displayed by any disk MBR analyzing tool, has been given below:
In the above information, the relative sector numbers for the starting of both partitions are 63 and 11277630 respectively, which are free from partitions and counted according to the number of sectors, available in disk.
|